Web (Transactions)
Explore how you can use our Direct API to offer Affirm as a payment option on your website. Learn more about the process and error handling.
Legacy Charges API
This integration uses the updated
v1/transactions
API endpoints. If you're using our legacyv2/charges
endpoints, see the legacy Web (Charges).
Overview
To start a payment with Affirm online, there are three main steps to complete:
- Create a checkout form.
- Securely tokenize the customer's information.
- Use the
checkout_token
to initiate the transaction.
The sections below walk you through the necessary steps to initiate a checkout.
Checkout Initiation
Initiate the Affirm application in four steps.
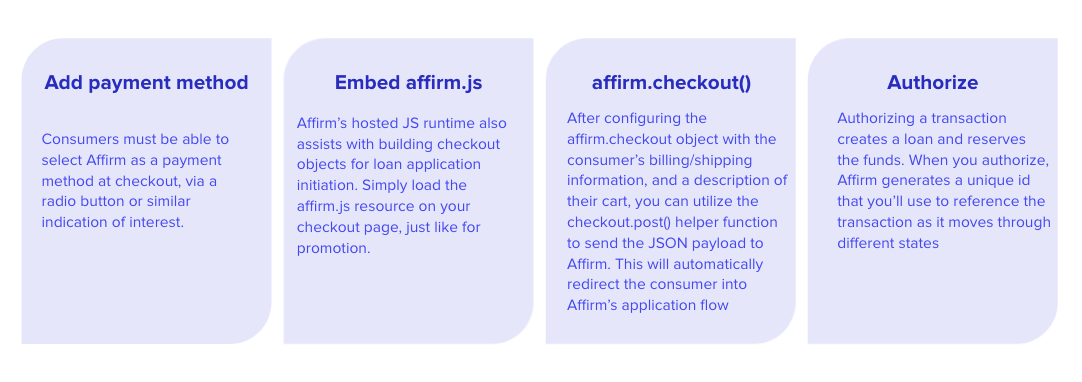
Checkout initiation occurs when the customer selects Affirm at checkout.
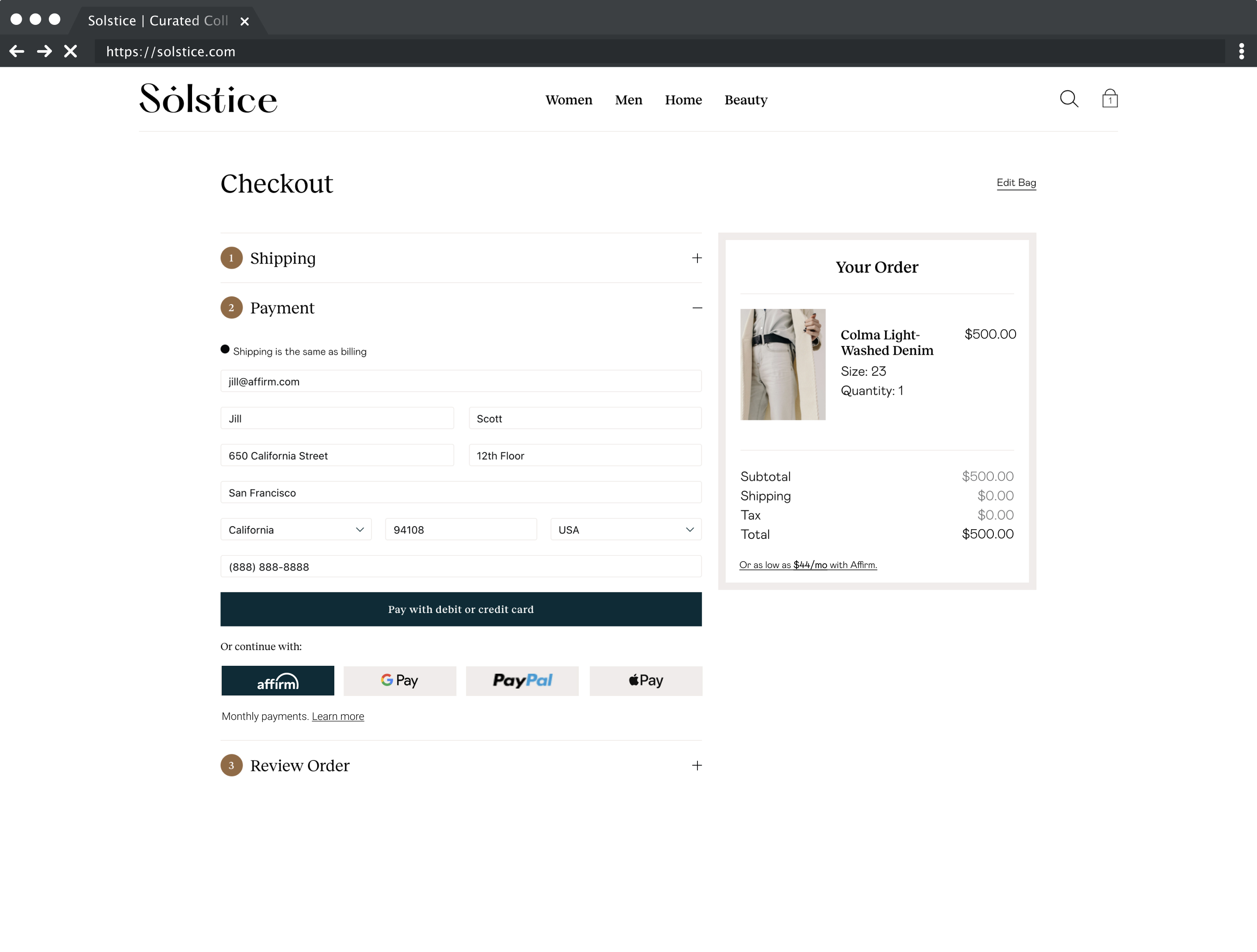
Checkout initiation example
Step 1. Embed Affirm.js
Account Setup
Make sure that you've set up an account in the Affirm Merchant Dashboard.
Include the following script in the head section on every page on your site. This script always loads directly from the Affirm domain.
<script>
var _affirm_config = {
public_api_key: "YOUR_PUBLIC_API_KEY", /* replace with public api key */
script: "https://cdn1-sandbox.affirm.com/js/v2/affirm.js",
locale: "en_US",
country_code: "USA",
};
(function(m,g,n,d,a,e,h,c){var b=m[n]||{},k=document.createElement(e),p=document.getElementsByTagName(e)[0],l=function(a,b,c){return function(){a[b]._.push([c,arguments])}};b[d]=l(b,d,"set");var f=b[d];b[a]={};b[a]._=[];f._=[];b._=[];b[a][h]=l(b,a,h);b[c]=function(){b._.push([h,arguments])};a=0;for(c="set add save post open empty reset on off trigger ready setProduct".split(" ");a<c.length;a++)f[c[a]]=l(b,d,c[a]);a=0;for(c=["get","token","url","items"];a<c.length;a++)f[c[a]]=function(){};k.async=
!0;k.src=g[e];p.parentNode.insertBefore(k,p);delete g[e];f(g);m[n]=b})(window,_affirm_config,"affirm","checkout","ui","script","ready","jsReady");
</script>
After you include the script above, a defined instance of Affirm is created on your client. You then gain access to methods that are within the Affirm object to trigger multiple actions.
Step 2. Render Affirm Checkout
When a customer chooses to use Affirm to pay for a purchase, a checkout
object containing the order details should be created so that Affirm can render the checkout flow. You can create a checkout
object within affirm.checkout()
and then launch the Affirm checkout experience using the affirm.checkout.open()
function.
You can configure the checkout object with the following:
- A user_confirmation_url to redirect your customer to a confirmation page after they confirm their loan
- A user_cancel_url to redirect your customer to a cancellation page if they don't complete their loan application.
- The customer's billing/shipping address.
- A description and total of their cart.
You can find more information about checkout object attributes in our checkout API.
affirm.checkout({
"merchant": {
"user_confirmation_url": "https://merchantsite.com/confirm",
"user_cancel_url": "https://merchantsite.com/cancel",
"public_api_key": "YOUR_PUBLIC_KEY",
"user_confirmation_url_action": "POST",
"name": "Your Customer-Facing Merchant Name"
},
"shipping":{
"name":{
"first":"Joe",
"last":"Doe"
},
"address":{
"line1":"633 Folsom St",
"line2":"Floor 7",
"city":"San Francisco",
"state":"CA",
"zipcode":"94107",
"country":"USA"
},
"phone_number": "4153334567",
"email": "[email protected]"
},
"billing":{
"name":{
"first":"Joe",
"last":"Doe"
},
"address":{
"line1":"633 Folsom St",
"line2":"Floor 7",
"city":"San Francisco",
"state":"CA",
"zipcode":"94107",
"country":"USA"
},
"phone_number": "4153334567",
"email": "[email protected]"
},
"items": [{
"display_name": "Awesome Pants",
"sku": "ABC-123",
"unit_price": 10000,
"qty": 3,
"item_image_url": "http://merchantsite.com/images/awesome-pants.jpg",
"item_url": "http://merchantsite.com/products/awesome-pants.html",
"categories": [
["Home", "Bedroom"],
["Home", "Furniture", "Bed"]
]
}
],
"discounts":{
"RETURN5":{
"discount_amount":500,
"discount_display_name":"Returning customer 5% discount"
},
"PRESDAY10":{
"discount_amount":1000,
"discount_display_name":"President's Day 10% off"
}
},
"metadata":{
"shipping_type":"UPS Ground",
"mode":"modal"
},
"order_id":"JKLMO4321",
"currency":"USD",
"financing_program":"flyus_3z6r12r",
"shipping_amount":1000,
"tax_amount":600,
"total":30100
})
affirm.checkout.open()
Calling the affirm.checkout()
function does the following:
- Sends the checkout object to the Affirm backend.
- Redirects the customer to the Affirm checkout process on the affirm.com domain or shows them an Affirm modal.
- Validates the required data in the checkout object
Step 3. Handle Callbacks
After you initiate a checkout and the customer confirms their Affirm loan, we send an HTTP request with the checkout_token
to the URL you defined in the checkout
object (user_confirmation_url). By default, Affirm sends this request via POST
. However, you can configure the checkout object to have Affirm send this request via GET
.
You choose how we send the checkout_token
by setting the user_confirmation_url_action
parameter in the checkout
object.
- Setting it to
POST
sends thecheckout_token
in the body of the HTTP request (default setting). - Setting it to
GET
sends thecheckout_token
in the query string of the HTTP request.
Modal Checkout
You can also retrieve the
checkout_token
from a javascript callback if you set the metadatamode
tomodal
. Learn more about modal checkout.
Step 4. Authorize
When a customer successfully completes a checkout, it is recorded as a new purchase attempt. This needs to be handled on your server-side to be fulfilled via our Transactions
API.
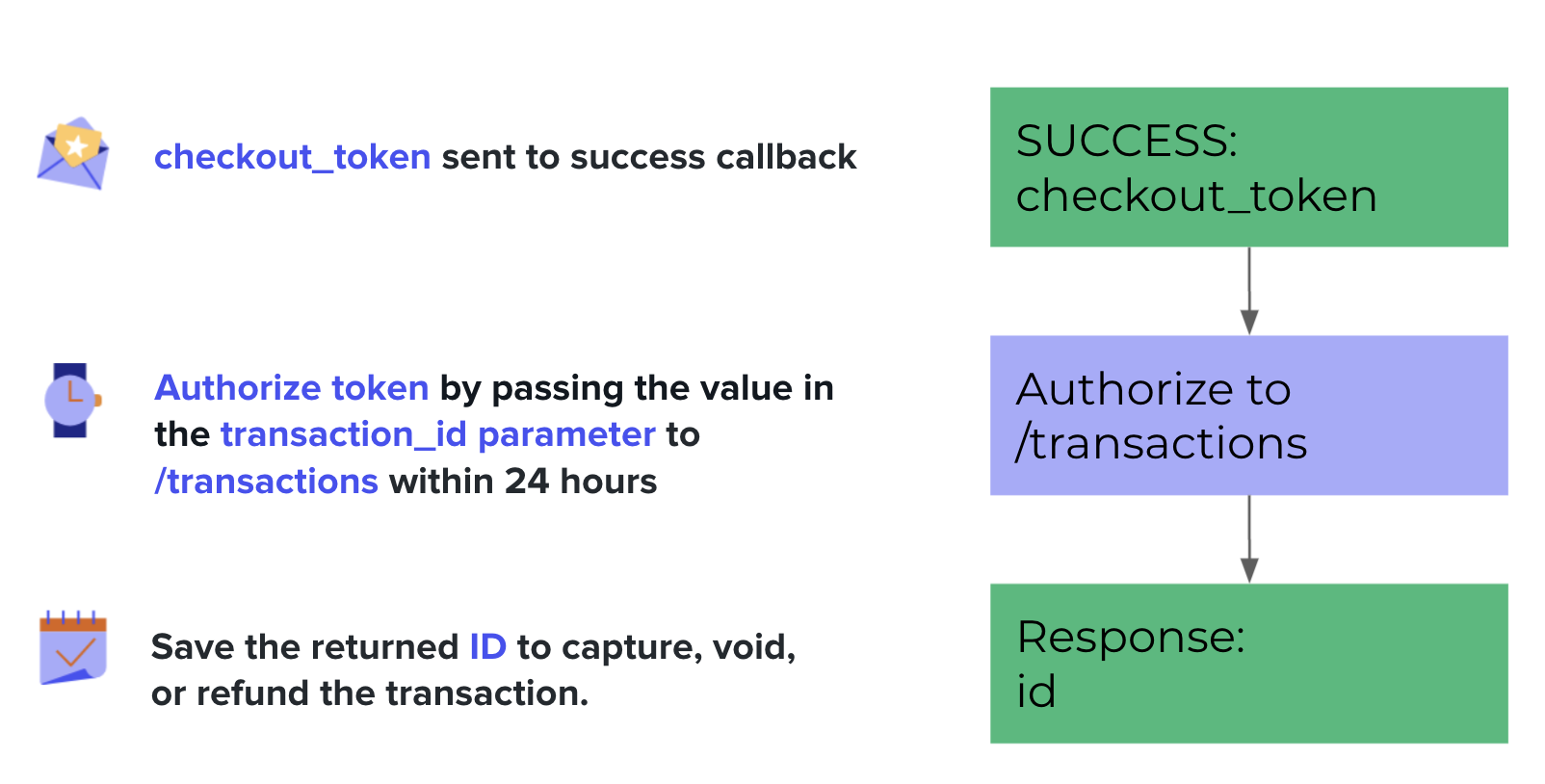
Authorizing an Affirm transaction.
1. Retrieve the checkout_token
checkout_token
Retrieve the checkout_token
sent by Affirm via a HTTP request to the success callback.
"checkout_token": "CJXXM8RERR0LC066"
2. Authorize the checkout_token
checkout_token
Authorize the checkout_token
immediately by passing the value in the transaction_id
parameter to /transactions
.
curl --request POST \
--url https://api.affirm.com/api/v1/transactions \
--header 'Accept: */*' \
--header 'Content-Type: application/json' \
--data '
{
"transaction_id": "CJXXM8RERR0LC066",
"order_id": "JKLM4321"
}
'
3. Save the returned id
After authorizing the transaction, save the id
from the transaction object returned in the response.
{
"id": "9RG3-PMSE"
"currency": "USD",
"amount": 11500,
}
Error Handling
Errors generated by the checkout request are presented on the page where checkout is initiated, in the form of a pop-up modal window. Specific messaging about the source of the error is presented in this modal (e.g., "Invalid phone number").
You may define a callback function when this error modal is closed, but currently that callback does not relay any event data specific to the error message that's displayed in the modal.
Here's an example of how this event callback would be defined:
affirm.ui.ready(
function() {
affirm.ui.error.on("close", function(){
alert("Please check your contact information for accuracy.");
});
}
);
Itinerary Object- Travel Merchant Requirement
If you are a travel merchant, you are required to include the itinerary object in your checkout flow. Learn more about the itinerary object.
Updated 2 months ago