Authorize and Capture Transactions
Learn about the authorization and capture flow.
Authorize transaction
The Authorize Transaction endpoint creates a loan and reserves the funds. When you authorize, Affirm will generate a unique id
that you’ll use to reference the transaction moving forward. You must authorize a transaction before you capture it.
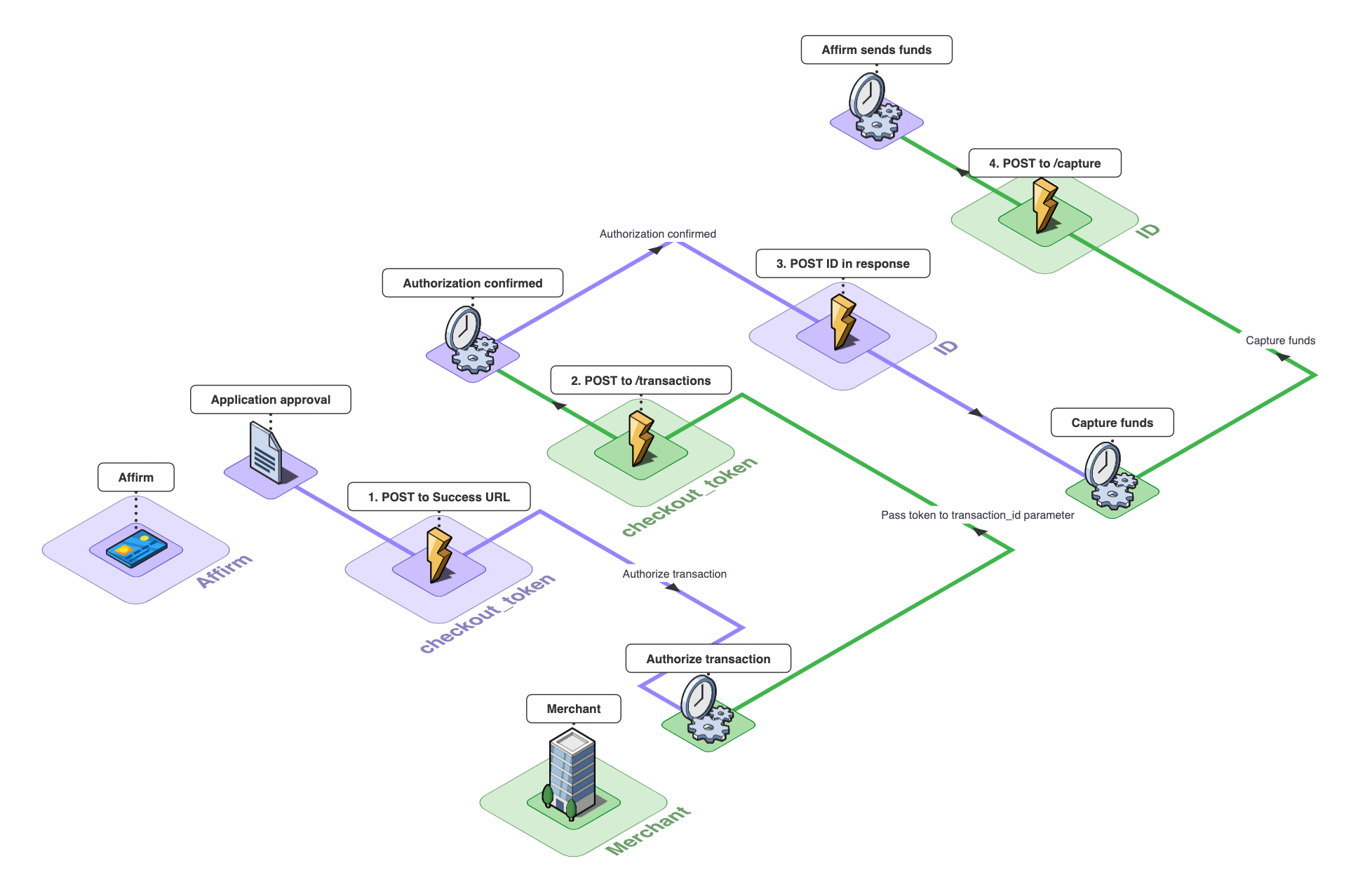
This represents the authorize and capture flow.
If you do not authorize a transaction, it will not be considered active. This means the user will not see the loan, and you will not be able to capture the funds. For this reason, you should authorize the loan as soon as you receive a
checkout_token
.
To authorize a transaction you'll need the checkout_token
returned from your Checkout Integration to pass it into the transaction_id
parameter.
curl https://sandbox.affirm.com/api/v1/transactions
-X POST
-u "{public_api_key}:{private_api_key}"
-H "Content-Type: application/json"
-d '{"transaction_id": "{checkout_token}","order_id": "{order_id}"}'
$endpoint = "https://sandbox.affirm.com/api/v1/transactions";
$data = '{"transaction_id": "' . $_POST["transactions_id"] . '"}';
try {
$response = callAffirm($endpoint, $data);
// Handle the response
} catch (Exception $e) {
// Handle the exception
}
using(var httpClient = new HttpClient()) {
using(var request = new HttpRequestMessage(new HttpMethod("POST"), "https://sandbox.affirm.com/api/v1/transactions")) {
var base64authorization = Convert.ToBase64String(Encoding.ASCII.GetBytes("<public_api_key>:<private_api_key>"));
request.Headers.TryAddWithoutValidation("Authorization", $ "Basic {base64authorization}");
request.Content = new StringContent("{\"transaction_id\":\"<checkout_token>\"}");
request.Content.Headers.ContentType = MediaTypeHeaderValue.Parse("application/json");
var response = await httpClient.SendAsync(request);
HttpContent responseContent = response.Content;
using(var reader = new StreamReader(await responseContent.ReadAsStreamAsync())) {
Console.WriteLine(await reader.ReadToEndAsync());
}
Console.ReadKey();
}
}
You should receive a response that contains a unique ID ("AMLC-5X0W" in this example).
{
"status": "authorized",
"amount_refunded": 0,
"provider_id": 1,
"created": "2021-06-23T23:25:55Z",
"order_id": "ABC123",
"checkout_id": "7WYDR0M83CGE47GJ",
"currency": "USD",
"amount": 49999,
"events": [
{
"currency": "USD",
"amount": 49999,
"type": "auth",
"id": "7WYDR0M83CGE47GJ",
"created": "2021-06-23T23:26:28Z"
}
],
"remove_tax": false,
"authorization_expiration": "2021-07-23T23:26:28Z",
"id": "AMLC-5X0W"
}
After successfully authorizing a transaction and receiving the response object, your site should do the following:
- Validate that the authorized amount equals the order total
- Store the
id
(transaction identifier) - Mark the order payment as pending
If the authorization fails, your site could potentially store this checkout attempt, as it is not required on our end.
You should only authorize a given Affirm loan once, for the entire amount of the
transaction being purchased. If you have a specific use case where this may be
difficult, please contact us at [email protected] or use the widget at the bottom of the page.
Capture a transaction
Once an order has been fulfilled, you must send a Capture API request to Affirm in order to capture or settle the funds. You will want to perform this activity from your secure back-end systems. To capture an authorized transaction, you'll need the id
provided in the Authorization
API response. There aren't any required fields that need to be stored from the Capture
response.
Capturing the funds is similar to capturing a credit card transaction. After you capture the loan, the following happens:
- Affirm notifies the customer that the loan has been captured and that their first payment is due to Affirm in 30 days (for monthly installments) or 2 weeks (for Pay in 4)
- Affirm pays the merchant within 2-3 business days via ACH transfer to the provided bank account
curl https://sandbox.affirm.com/api/v1/transactions/{id}/capture
-X POST
-u {public_api_key}:{private_api_key}"
-H "Content-Type: application/json"
-d '{"order_id": "{order_id}", "shipping_carrier": "USPS", "shipping_confirmation": "1Z23223", "amount":49999}'
$endpoint = "https://sandbox.affirm.com/api/v1/transactions";
$url = $endpoint . "/" . $_GET["id"] . "/capture";
$data = '';
try {
$response = callAffirm($url, $data);
// Handle the response
} catch (Exception $e) {
// Handle the exception
Note that you can perform a partial capture by specifying a value in cents within the
amount
parameter.
The cumulative sum of partial capture amounts can not exceed the originally authorized total.
You will then receive a response with the confirmation.
{
"fee": 1500,
"created": "2021-06-23T23:27:13Z",
"order_id": "ABC123",
"currency": "USD",
"amount": 49999,
"reference_id": "6789",
"type": "capture",
"id": "7WYDR0M83CGE47GJ"
}
Updated 14 days ago